GitHub Copilot is a programming assistant that uses AI to suggest code in Visual Studio Code. It analyzes your project context to provide relevant functions or line recommendations that you can accept, edit, or reject. Copilot learns your preferences and style over time.
This guide explains how to use GitHub Copilot and leverage its auto-complete capabilities.
Install and configure GitHub Copilot
To use GitHub Copilot, first install Visual Studio Code – Microsoft’s free cross-platform code editor. Once you have VS Code, download the Copilot extension from its Marketplace.
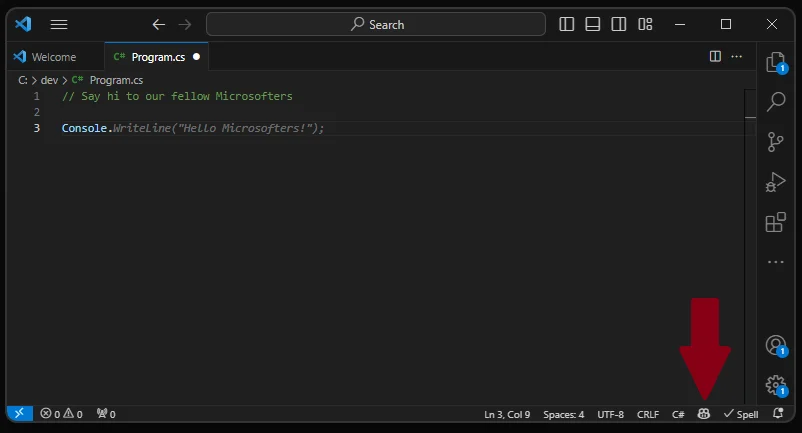
“After Installation, Access Copilot Options via the VS Status Bar Icon”
Use Copilot to write code
“Copilot can help with lots of programming languages like Python, JavaScript, Java, C#, Ruby, PHP, or Go. To use it, just make or open a file with the right language ending, and start typing code.
When you’re typing, Copilot pops up with suggestions in a little window. You can say yes to a suggestion by hitting the Tab key, or no by pressing Esc. You can move through suggestions with the arrow keys, or see more options by clicking the three-dot icon.”
Here are some examples of code generated by Copilot for different languages:
Python
# create a function that adds two numbers
def add(a, b):
return a + b
JavaScript
// sort the array in ascending order
let array = (5, 2, 7, 4, 1, 6, 3);
array.sort((a, b) => a - b);
Java
// create a class that represents a person
public class Person {
private String name;
private int age;
// constructor
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// getter and setter methods
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
Advanced features of GitHub Copilot
“Copilot does more than just suggest lines of code. It can create entire features, write documentation, make tests, or add comments for you. All you have to do is write the name of what you need, like a function or a comment, and leave a blank line below it. Copilot will do its best to finish the rest for you.”
Here are some examples of Copilot’s advanced features:
Python
# write a function that reverses a string
def reverse_string(s):
# initialize an empty string
reversed = ""
# loop through the characters of the original string in reverse order
for i in range(len(s) - 1, -1, -1):
# append each character to the reversed string
reversed += s(i)
# return the reversed string
return reversed
JavaScript
/**
* Write a documentation for this function
* @param {number} x - the first number
* @param {number} y - the second number
* @returns {number} - the sum of x and y
*/
function add(x, y) {
return x + y;
}
Java
// write a test for the Person class
import org.junit.Test;
import static org.junit.Assert.*;
public class PersonTest {
@Test
public void testPerson() {
// create a person object with name Alice and age 25
Person person = new Person("Alice", 25);
// check if the name and age are correct
assertEquals("Alice", person.getName());
assertEquals(25, person.getAge());
}
}
C#
// write a comment that explains what this code does
// this code creates a list of numbers from 1 to 10 and prints them
List<int> numbers = new List<int>();
for (int i = 1; i <= 10; i++)
{
numbers.Add(i);
}
foreach (int number in numbers)
{
Console.WriteLine(number);
}
“GitHub Copilot is an amazing tool that can make coding easier and quicker. Give it a try and explore its many features! To learn more about Copilot, check out its official website or documentation.
I hope you found this tutorial helpful and learned something new. If you enjoyed it, feel free to share it with your friends and leave a comment with your thoughts. Until next time, happy coding!”